Binding mouse events for range inputs with Knockout js
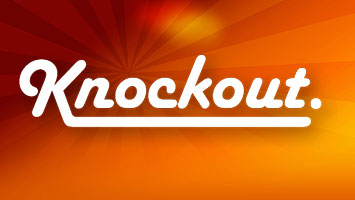
HTML5 range input is a great control and an alternative way for a user to select or set a value. Knockout is a great fit for handling it.
Recently I ran across a problem when using a range input with Knockout. I had a requirement to handle mousedown
and mouseup
events. Knockout allows you to add an event handler with the event binding, so we can do something like this.
Example:
<input type="range" min="1" max="10" data-bind="event: { mousedown: mouseDown, mouseup: mouseUp }">
<script type="text/javascript">
var viewModel = {
mouseDown: function() {
console.log('mousedown event fired');
},
mouseUp: function() {
console.log('mouseup event fired');
}
};
ko.applyBindings(viewModel);
</script>
The events fire as expected but the range slider does not move. mousedown
is actually blocking anything else from happening here. We need to return true
for the event to complete.
var viewModel = {
mouseDown: function() {
console.log('mousedown event fired');
return true;
},
mouseUp: function() {
console.log('mouseup event fired');
}
};
ko.applyBindings(viewModel);
The slider moves perfectly but mouseup
is blocking and we can't let go of the slider!
var viewModel = {
mouseDown: function() {
console.log('mousedown event fired');
return true;
},
mouseUp: function() {
console.log('mouseup event fired');
return true;
}
};
ko.applyBindings(viewModel);
Now the range input works as expected and we can handle the mouse events using Knockout.