Clean up your views with display templates - MVC
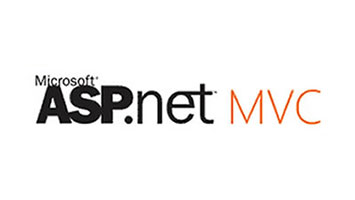
Looping through lists is not uncommon in views but it can cause bloat and just look plain ugly. Display templates solve this.
I recently blogged about why I think display templates are awesome. My number one win is replacing nasty foreach
loops with a display template.
Example
Let's use a blog landing page for this example. My view model has provided me with a list of recent posts, a list of categories and a list of tags. My view would typically look something like this.
@model PostsViewModel
<h1>Recent posts</h1>
<ol>
@foreach (var post in Model.Posts)
{
<li>
<a href="@post.Url">@post.Title</a>
</li>
}
</ol>
<aside>
<h2>Categories</h2>
<ol>
@foreach (var category in Model.Categories)
{
<li>
<a href="@category.Url">
@category.Name <span>@category.Count</span>
</a>
</li>
}
</ol>
<h2>Tags</h2>
<ol>
@foreach (var tag in Model.Tags)
{
<li>
<a href="@tag.Url">@tag.Name</a>
</li>
}
</ol>
</aside>
Straight away we can see these foreach
statements are bloating the view. We could put these into partial views, this would make the view cleaner, but we would still have those loops. With display templates we can make it cleaner still by getting rid of the loops.
How come?
We can pass a model or a list of models into Html.DisplayFor()
and the framework will take care of the rest. These are our display templates:
RecentPost.cshtml
<li>
<a href="@post.Url">@post.Title</a>
</li>
Category.cshtml
<li>
<a href="@category.Url">
@category.Name <span>@category.Count</span>
</a>
</li>
Tag.cshtml
<li>
<a href="@post.Url">@post.Title</a>
</li>
Leaving us with a nice clean view.
@model PostsViewModel
<h1>Recent posts</h1>
<ol>
@Html.DisplayFor(x => x.Posts)
</ol>
<aside>
<h2>Categories</h2>
<ol>
@Html.DisplayFor(x => x.Categories)
</ol>
<h2>Tags</h2>
<ol>
@Html.DisplayFor(x => x.Tags)
</ol>
</aside>
Summary
Display templates are a useful HTML helper built into the MVC framework. Using them to replace foreach
loops allows us to write clean, readable code that can easily be used across the application. Start removing your loops today!