Request task cancellation extension method
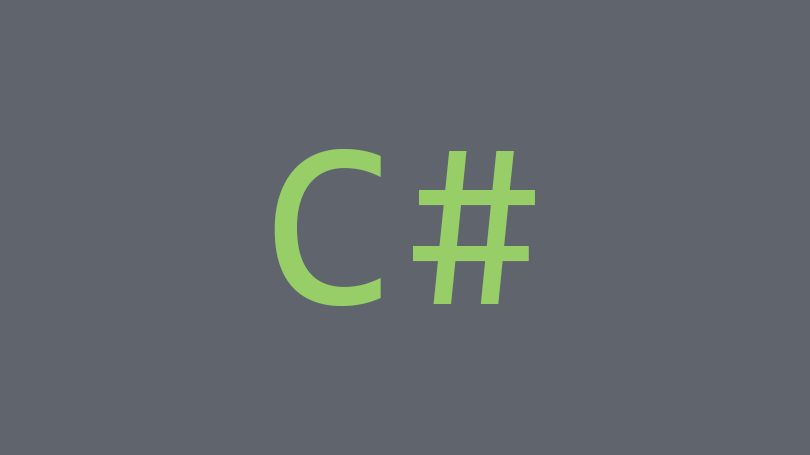
An extension method to request the cancellation of a CancellationTokenSource
.
A common pattern I came across when writing async methods that could be cancelled with a CancellationTokenSource
was:
if (this.cancellationTokenSource != null && !this.cancellationTokenSource.IsCancellationRequested)
{
this.cancellationTokenSource.Cancel();
}
Instead of repeating the code across the project I wanted to encapsulate this into a method, extending the CancellationTokenSource
class. So I refactored the code into an extension method.
public static class CancellationTokenSourceExtensions
{
public static void CancelIfCancellable(this CancellationTokenSource ctx)
{
if (ctx != null && !ctx.IsCancellationRequested)
{
ctx.Cancel();
}
}
}
This can be called like so.
this.cancellationTokenSource.CancelIfCancellable();
Now our methods are much cleaner and simpler. Win!